HTTP Event Sources
HTTP Event Source allows applications to send event data to the messaging platform via API requests. Unlike JavaScript Event Sources, HTTP Event Sources do not rely on client-side scripts. Instead, they enable backend systems, third-party applications, or other servers to transmit event data through HTTP requests.
Use HTTP Event Sources as an example to:
Log user sign-ups or transactions from a backend system.
Send event notifications when specific actions occur, such as order fulfillment.
Track mobile app interactions, where JavaScript-based tracking is not applicable.
Capture system-generated alerts from IoT devices or cloud services.
In many cases, when a server-side event occurs, you may want to trigger an automated response. HTTP Event Sources enable seamless integration of real-time event tracking, allowing backend systems to process events dynamically and trigger workflows efficiently.
Add & Configure HTTP Event Source
Follow the steps below to create and configure HTTP Event Source.
Step 1: Create HTTP Event Source
Follow these steps to create an HTTP Event Source:
Navigate to Automations -> Event Sources.
Click Add Event Source and select HTTP.
Enter a Name for your event source and click OK.
Once created, the Overview section will display:
API Key (needed for authentication).
Default Status: No activity (indicating no events have been received yet).
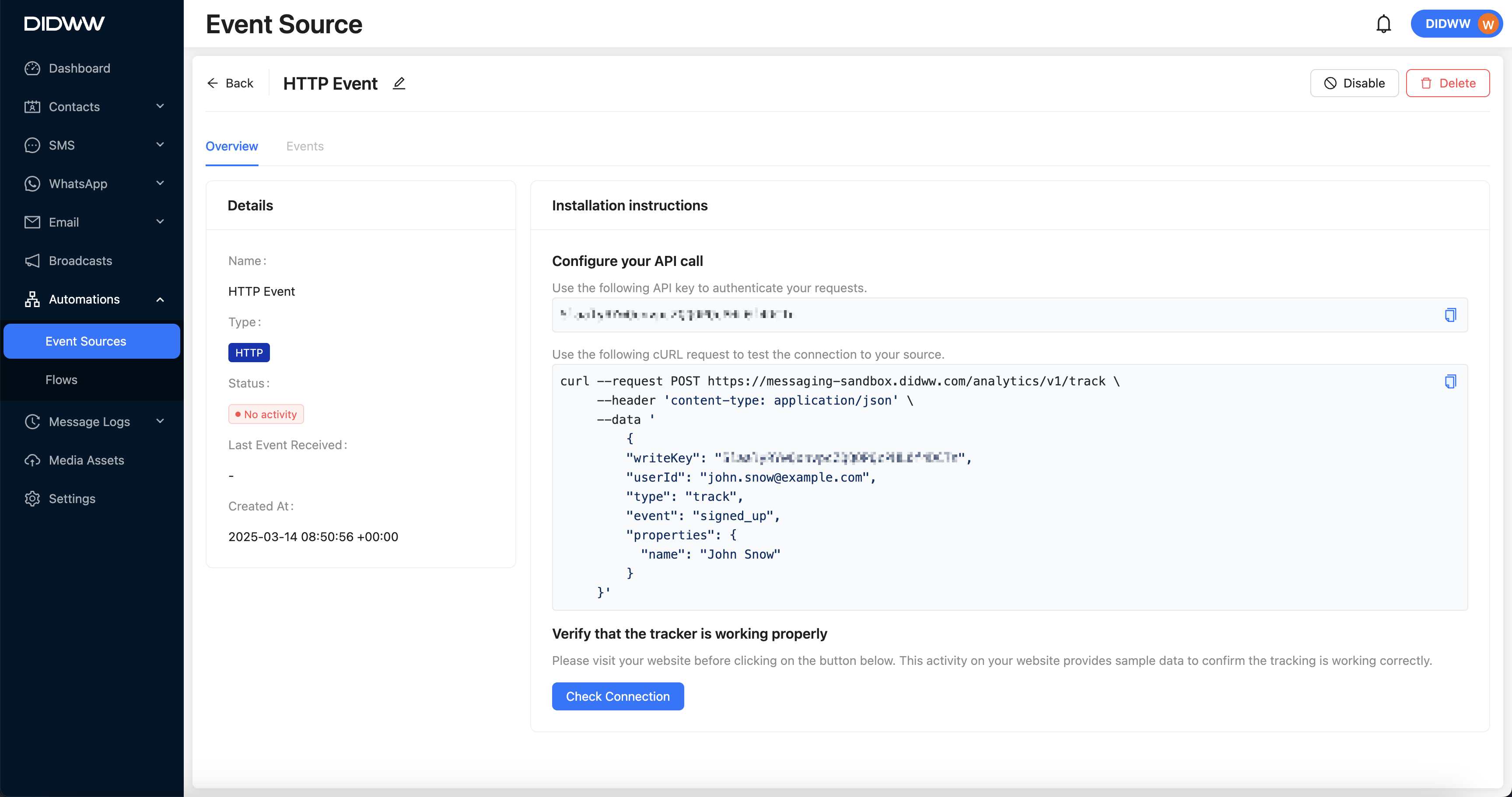
Fig. 1. Create HTTP Event Flow Overview.
Step 2: Configure Your API Call
To send event data to your HTTP Event Source, use the provided API Key in your HTTP request.
Use the method that best fits your use case. For example, you can send event data to your HTTP Event Source using one of the following methods:
cURL (Command Line Request) – Send a request from a terminal or command prompt.
Postman (API Testing Tool) – Test and send requests using a user-friendly interface.
Backend Integration (Python, Node.js, Java, etc.) – Integrate event tracking into your backend system.
Web Requests – Send events from a web-based frontend.
Note
If you copy the snippet examples from the documentation page, instead of the messaging platform, replace
YOUR_API_KEY
with the actualwriteKey
provided in your Event Source configuration.The
writeKey
is unique per event source and is required for data tracking.
Using cURL (Command Line Request)
Run the following cURL command in a terminal (macOS/Linux) or PowerShell (Windows):
curl --request POST https://messaging-sandbox.didww.com/analytics/v1/track \ --header 'content-type: application/json' \ --data ' { "writeKey": "YOUR_API_KEY", "userId": "john.snow@example.com", "type": "track", "event": "signed_up", "properties": { "name": "John Snow" } }'
Using Postman (API Testing Tool)
Open Postman and set the request type to POST.
Enter the URL from snippet code example: https://messaging-sandbox.didww.com/analytics/v1/track
In the Headers section, add:
Content-Type: application/json
In the Body section, choose raw and paste the following JSON data:
{ "writeKey": "YOUR_API_KEY", "userId": "john.snow@example.com", "type": "track", "event": "signed_up", "properties": { "name": "John Snow" } }Click Send to test the request.
Using Python (Requests Library)
To send event data using Python use the following example:
import requests url = "https://messaging-sandbox.didww.com/analytics/v1/track" headers = {"Content-Type": "application/json"} data = { "writeKey": "YOUR_API_KEY", "userId": "john.snow@example.com", "type": "track", "event": "signed_up", "properties": {"name": "John Snow"} } response = requests.post(url, json=data, headers=headers) print(response.status_code, response.json())
Step 3: Verify the Event Source Connection
After sending the test request, follow these steps to verify that the HTTP Event Source is receiving data:
Click the Check Connection button in the Event Source Overview section.
If the event is successfully received, the Status will change:
If the event is not received, the status remains as no activity, and the following message appears: “Source is not connected. We haven’t received any data. Please verify that the Source is installed correctly.”
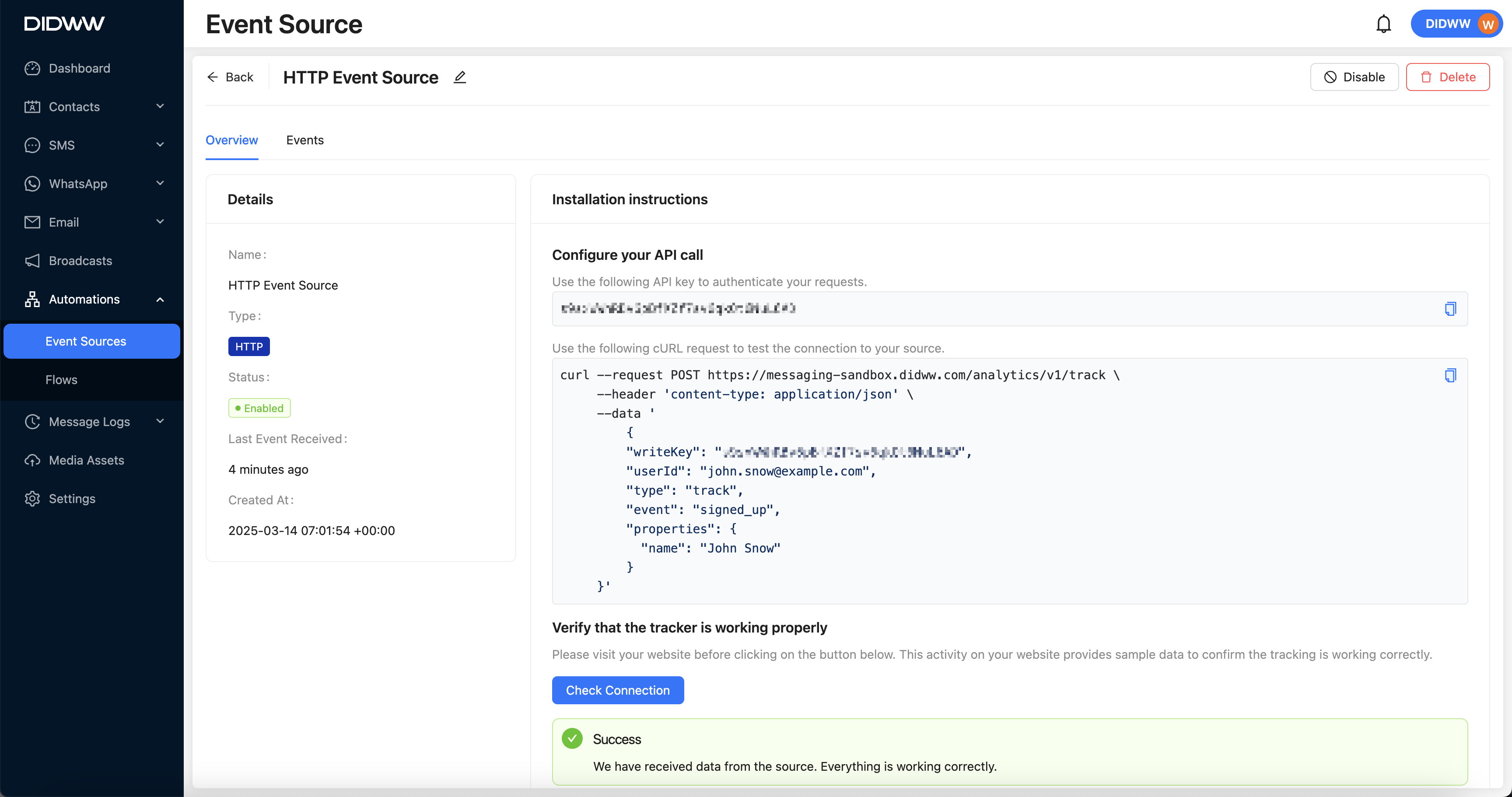
Fig. 1. Successful HTTP Event Source Connection.
Event Source Statuses
HTTP Event Source can have one of the following statuses:
Viewing HTTP Events
To view the events associated with a source, follow the steps below:
Go to Automations > Event Sources.
Select the event source, click the
button, and choose Details, or click the source name.
In the Details page, click the Events tab. If events were sent, the latest event appears at the top.
Click an event to expand it and view additional details, including attributes that were delivered.
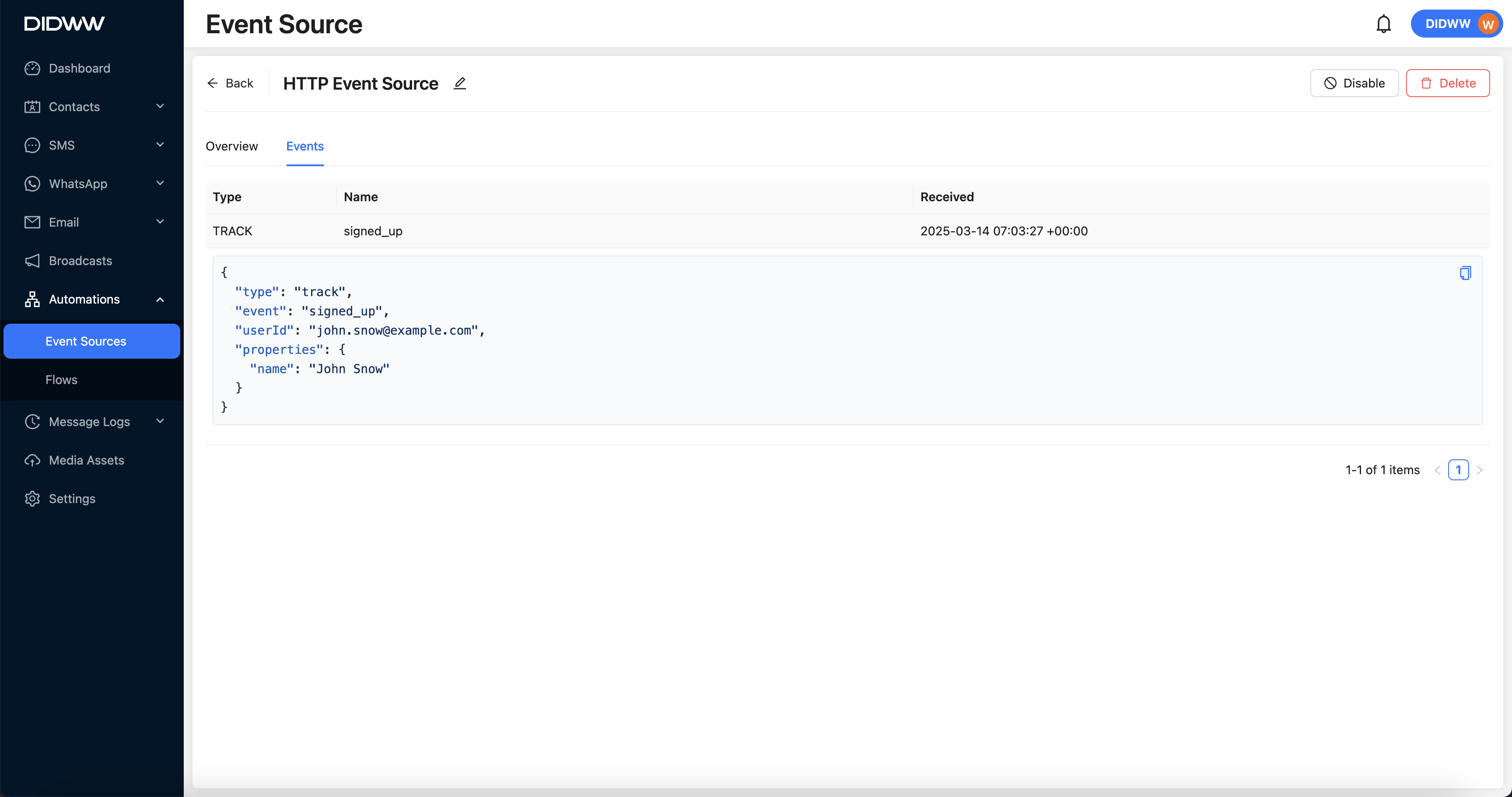
Fig. 2. Viewing events.
HTTP Event Source API endpoints
The following are different event types that can be tracked in your system. These events help categorize and track user actions, page views, and user data:
Note
The HTTP Endpoints support only the
POST
method.The following examples are using a placeholder WriteKey. Make sure that you use your WriteKey to perform successful
Post
API calls.The only mandatory body field is
"writeKey": "your_writeKey_value"
, all other fields are optional based on your configuration.
Supported HTTP Event Types
Nr.
Event Type
Description
1
Logs specific actions or events, such as sign-ups, purchases, or button clicks.
2
Tracks when a user views a webpage, capturing details like URL and title.
3
Identifies or updates user information, such as name or email.
4
Logs screen views within a mobile application, similar to the
Page
event.5
Links an anonymous user session to a known user by assigning a new ID.
6
Tracks group-level data, such as teams, organizations, or collective entities.
Event Type Details & Examples
Each HTTP Event Type allows you to track and categorize different kinds of user interactions or data within your system. The type of event determines what information is captured and how it is processed.
1. HTTP event type: Track
The Track
endpoint is used to log specific actions or events performed by a user, such as signing up, clicking a button, or making a purchase.
Example Use Case: Track when a user signs up or makes a purchase.
http
POST /analytics/v1/track HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "track", "event": "signed_up", "properties": { "name": "John Snow" } }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/track -H "Content-Type: application/json" --data-raw '{"event": "signed_up", "properties": {"name": "John Snow"}, "type": "track", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
2. HTTP event type: Page
The Page
endpoint tracks when a user views a page on a website or application. This event typically contains information about the page visited (URL, title, etc.).
Example Use Case: Track page views like a user visiting a product page.
http
POST /analytics/v1/page HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "page", "name": "Product Page", "properties": { "url": "https://example.com/product/12345" } }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/page -H "Content-Type: application/json" --data-raw '{"name": "Product Page", "properties": {"url": "https://example.com/product/12345"}, "type": "page", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
3. HTTP event type: Identify
The Identify
endpoint is used to identify or update a user. This event can include any user-specific data like name, email, or other attributes.
Example Use Case: Use this event to update a user’s profile or track new user data.
http
POST /analytics/v1/identify HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "identify", "traits": { "name": "John Snow", "email": "john.snow@example.com" } }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/identify -H "Content-Type: application/json" --data-raw '{"traits": {"email": "john.snow@example.com", "name": "John Snow"}, "type": "identify", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
4. HTTP event type: Screen
The Screen
endpoint is used in mobile apps to track when a user views a specific screen within the app. It’s similar to Page events but specific to mobile.
Example Use Case: Track which screen the user is currently viewing in a mobile app.
http
POST /analytics/v1/screen HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "screen", "name": "Home Screen", "properties": { "device": "iPhone 16" } }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/screen -H "Content-Type: application/json" --data-raw '{"name": "Home Screen", "properties": {"device": "iPhone 16"}, "type": "screen", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
5. HTTP event type: Alias
The Alias
endpoint is used to assign a new ID to an existing user or link anonymous data to a known user. This is useful when transitioning from an anonymous session to a known user.
Example Use Case: Link an anonymous session to a user after they sign up or log in.
http
POST /analytics/v1/alias HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "alias", "previousId": "anon12345" }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/alias -H "Content-Type: application/json" --data-raw '{"previousId": "anon12345", "type": "alias", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
6. HTTP event type: Group
The Group
endpoint type is used to track group-level data. This can refer to teams, organizations, or other collective entities a user belongs to.
Example Use Case: Track information related to a user’s organization or team.
http
POST /analytics/v1/group HTTP/1.1 Host: messaging.didww.com Content-Type: application/json { "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y", "userId": "john.snow@example.com", "type": "group", "groupId": "company12345", "traits": { "name": "Example Corp", "industry": "Technology" } }curl
curl -i -X POST https://messaging.didww.com/analytics/v1/group -H "Content-Type: application/json" --data-raw '{"groupId": "company12345", "traits": {"industry": "Technology", "name": "Example Corp"}, "type": "group", "userId": "john.snow@example.com", "writeKey": "a7BcG9tD3Xw0lNk1JqZ5YpQ9A2V8uF3Y"}'
Additional Resources
Learn how to automate messaging workflows using predefined triggers.
Read the API documentation for Event Sources HTTP integration, including setup and request structure.